Secure Authentication and Authorization Between Laravel Microservices Using JWT Tokens
In the world of microservices architecture, managing user authentication and authorization is crucial. JSON Web Tokens (JWT) provide a secure and efficient way to handle these requirements. This guide will walk you through using JWT tokens for authentication and authorization between Laravel microservices, with detailed explanations and code examples.
- Last Update: 2024/09/26
- Beginner to Advanced
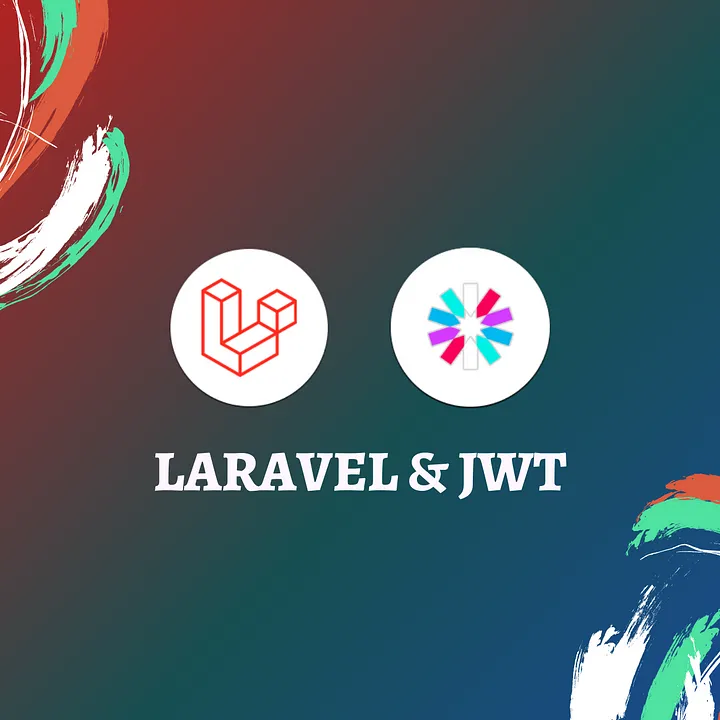
1. Introduction to JWT
What is JWT?
JSON Web Token (JWT) is a compact, URL-safe token format used to securely transmit information between parties. It consists of three parts:
- Header: Contains metadata about the token, including the type of token and the signing algorithm.
- Payload: Contains the claims or data to be transmitted, such as user information or permissions.
- Signature: Used to verify that the sender of the JWT is who it says it is and to ensure that the message wasn’t changed along the way.
Key Benefits of JWT
- Stateless: JWTs are self-contained and do not require server-side session storage. This statelessness is ideal for distributed systems like microservices.
- Secure: JWTs can be signed to ensure data integrity and optionally encrypted to ensure confidentiality.
- Scalable: JWTs facilitate horizontal scaling by allowing different services to verify tokens independently without relying on centralized session storage.
2. Setting Up Laravel Microservices
In this setup, we have two Laravel microservices:
- AuthService: Manages user authentication and issues JWT tokens.
- CRMService: Manages customer relationship data and uses JWT tokens for authorization.
Prerequisites
Ensure that Laravel is installed and set up for both microservices. Basic knowledge of Laravel and REST APIs is required.
3. Installing and Configuring Laravel Passport
Laravel Passport provides OAuth2 server functionality, including JWT support. Here’s how to set it up:
Step 1: Install Laravel Passport
Install Passport via Composer in both AuthService and CRMService:
Step 2: Publish Passport Configurations
Run the following command to create Passport’s encryption keys and migration files:
This command generates the keys necessary for signing tokens and the initial database migrations for Passport.
Step 3: Set Up Passport in AuthService
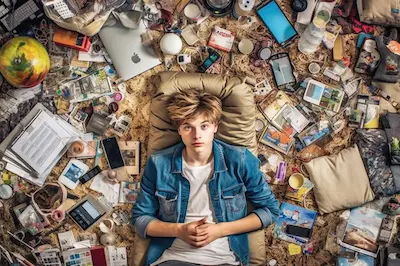
Add HasApiTokens Trait
In the User model, add the HasApiTokens trait provided by Passport. This trait enables the model to issue and manage API tokens.
Register Passport Routes
In the AuthServiceProvider, add Passport routes to handle token issuance and validation:
Configure API Guard
In config/auth.php, configure the API guard to use Passport. This tells Laravel to use Passport’s token driver for API authentication.